`React.StrictMode` 是一個好用的元件,用於突出顯示應用程式中的潛在問題。就像 `<Fragment>` 一樣,`<StrictMode>` 不會渲染任何額外的 DOM 元素。在這篇文章中,我們將深入探討什麼是嚴格模式、它是如何運作的,以及為什麼你應該考慮使用看看。 原文出處:https://dev.to/codeofrelevancy/what-is-strict-mode-in-react-3p5b --- ## 什麼是 React 中的嚴格模式? 嚴格模式是一組開發工具,可幫助您在程式碼中找到潛在問題。當您在 React 應用程式中啟用嚴格模式時,您實際上是在告訴 React 打開一堆額外的檢查👀 和警告,以便幫助您寫出更好的程式碼。這些檢查和警告可以捕獲以下內容: 1. 有副作用的元件 2. 已棄用或不安全的生命週期方法 3. 某些內置函數的不安全用法 4. 列表中的重複鍵 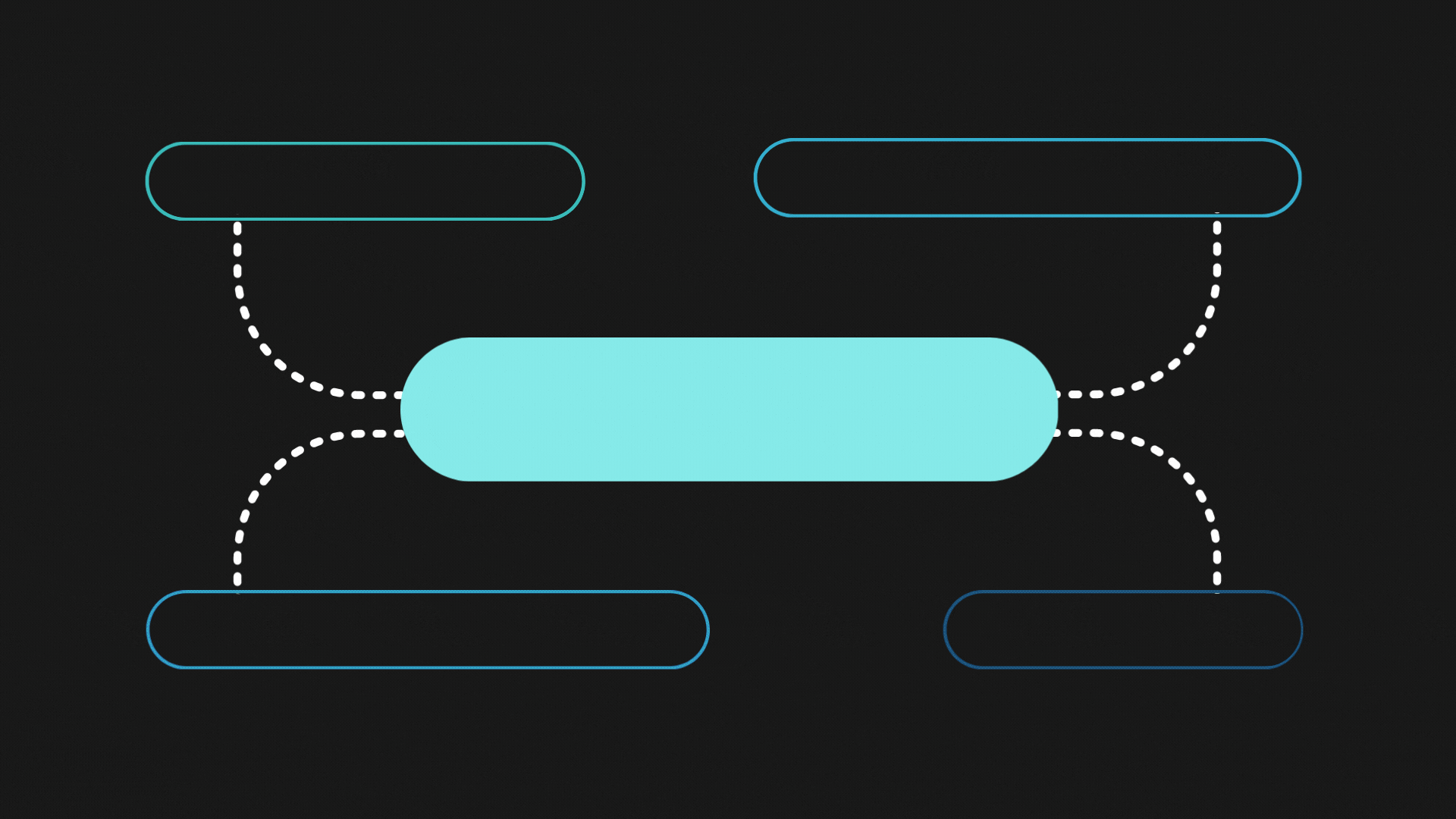 --- ## 啟用嚴格模式 在 React 應用程式中啟用嚴格模式非常簡單。您可以通過在主 `index.js` 文件中加入一行程式碼來實現: ``` import React from 'react'; import ReactDOM from 'react-dom'; ReactDOM.render( <React.StrictMode> <App /> </React.StrictMode>, document.getElementById('root') ); ``` ## 為應用程式的一部分啟用嚴格模式 您還可以為應用程式的任何部分啟用嚴格模式: ``` import { StrictMode } from 'react'; function App() { return ( <> <Header /> <StrictMode> <main> <Sidebar /> <Content /> </main> </StrictMode> <Footer /> </> ); } ``` 在這種情況下,嚴格模式檢查不會針對“頁首”和“頁腳”元件執行。但是,它們將在 `Sidebar` 和 `Content` 以及它們內部的所有元件上執行,無論它有多深層。 --- ## 嚴格模式只影響開發環境 請務必注意,嚴格模式對 React 應用程式的正式環境建置沒有影響。嚴格模式啟用的任何檢查或警告都不會出現在用戶看到的應用程式最終版本中。除此之外,嚴格模式會打開額外的檢查和警告,它可能會減慢您的開發環境速度。 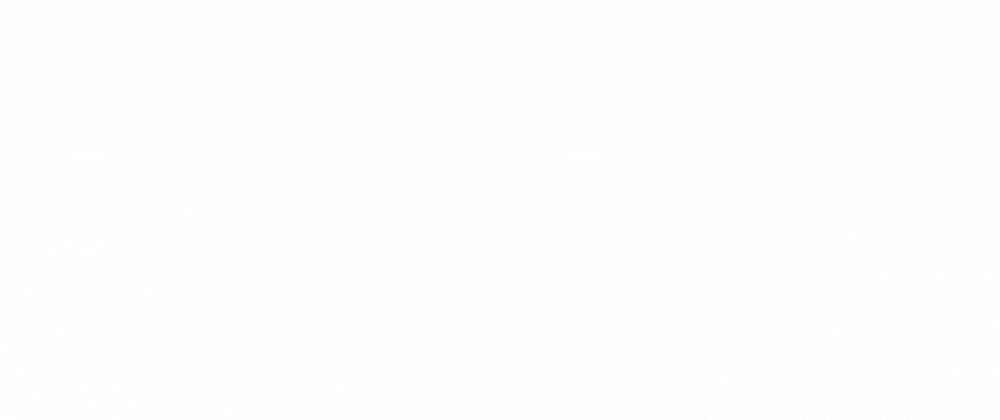 --- ## 嚴格模式可以幫助你捕捉細微的錯誤 有時 React 應用程式中的錯誤可能很難追踪,特別是諸如 race conditions 或對元件狀態的不正確假設等細微問題。啟用嚴格模式的額外檢查和警告,您可以在這些錯誤導致嚴重問題之前發現它們。 --- ## 嚴格模式可以幫助您了解最新的最佳實踐 React 是一個快速發展的框架,最佳實踐會隨著時間而改變。啟用嚴格模式並留意它提供的警告和建議,您可以確保您的 React 程式碼遵循當前的最佳實踐。例如在渲染列表時使用 key 屬性,或者在 render() 中避免副作用。 --- ## 儘早發現潛在問題 嚴格模式可以在您的程式碼中發現可能在未來引起的問題,以免它們成為嚴重問題。比方說,它可以檢測並警告已棄用的生命週期方法以及對不再推薦的 [findDOMNode()](https://react.dev/reference/react-dom/findDOMNode) 的存取。 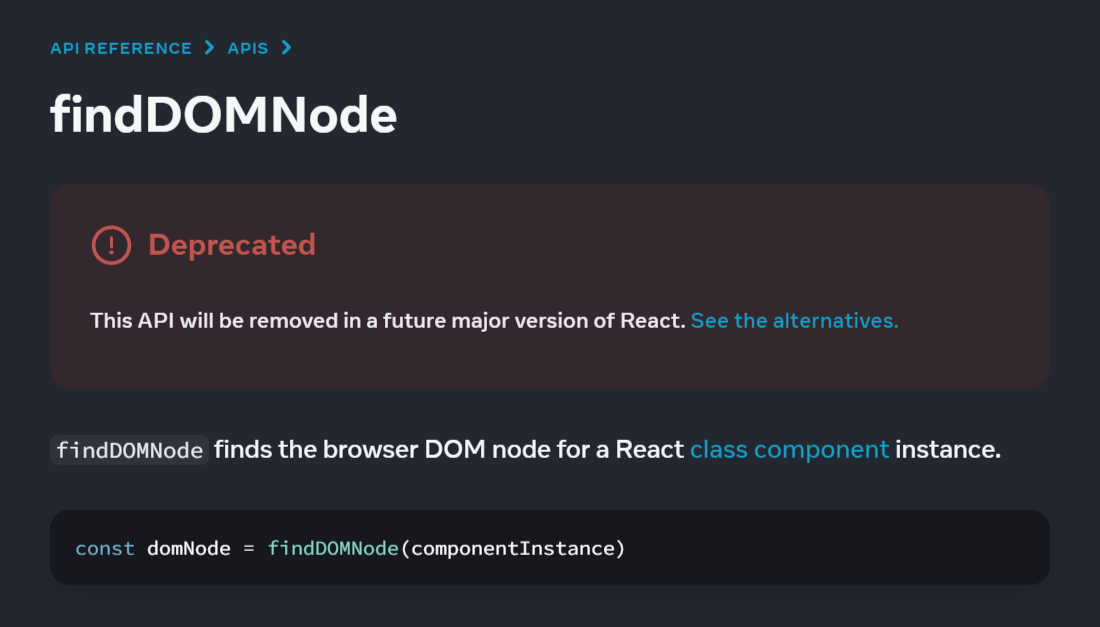 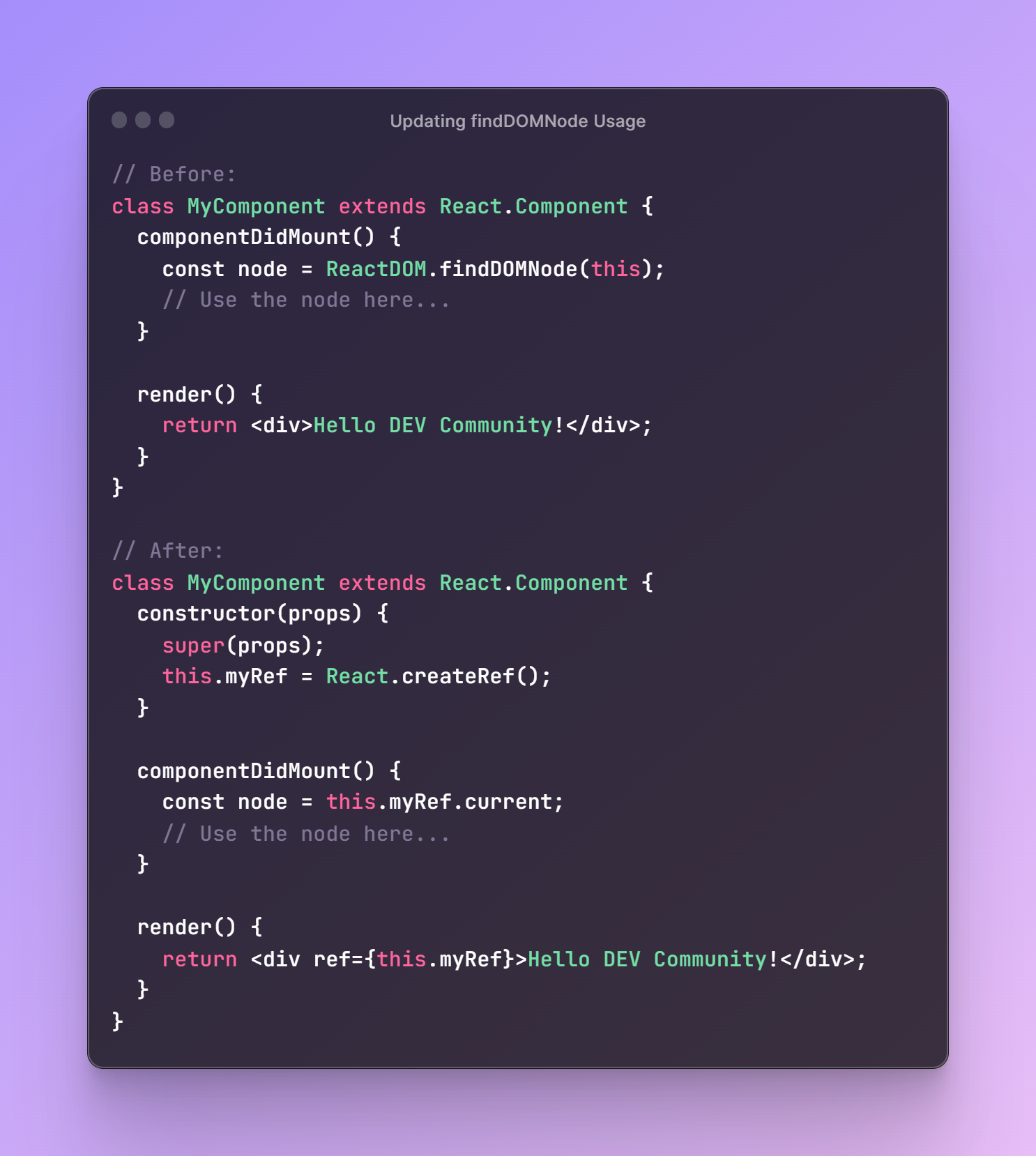 --- ## 防止常見錯誤 啟用嚴格模式,您可以避免一些隱晦的常見錯誤,例如:直接修改狀態而不是使用 setState()、使用未宣告變數。 --- ## 辨識不安全的生命週期 React Strict Mode 可以幫助辨識元件中不安全生命週期方法的使用。例如,它可以警告您使用 `componentWillUpdate` 和 `componentWillReceiveProps` 方法,這兩種方法都被認為是不安全的,將在 React 的未來版本中被刪除。  假設您有一個元件,它使用 `componentWillReceiveProps()` 來根據傳入的 props 更新其狀態: ``` class BlackMamba extends React.Component { constructor(props) { super(props); this.state = { venom: props.venom }; } componentWillReceiveProps(nextProps) { this.setState({ venom: nextProps.venom }); } render() { return <div>{this.state.venom}</div>; } } ``` 在常規模式下,該元件會按預期工作,但在“React.StrictMode”下,您會在控制台中看到一條警告: > 警告:BlackMamba 使用 `componentWillReceiveProps`,這被認為是一種不安全的生命週期方法。請使用靜態的 getDerivedStateFromProps 。 --- ## 過時的字串引用 API 用法 嚴格模式還可以警告您過時的字串 `ref` API 的使用。該 API 已被官方棄用。您應該使用更安全、更易於使用的 `createRef` API。 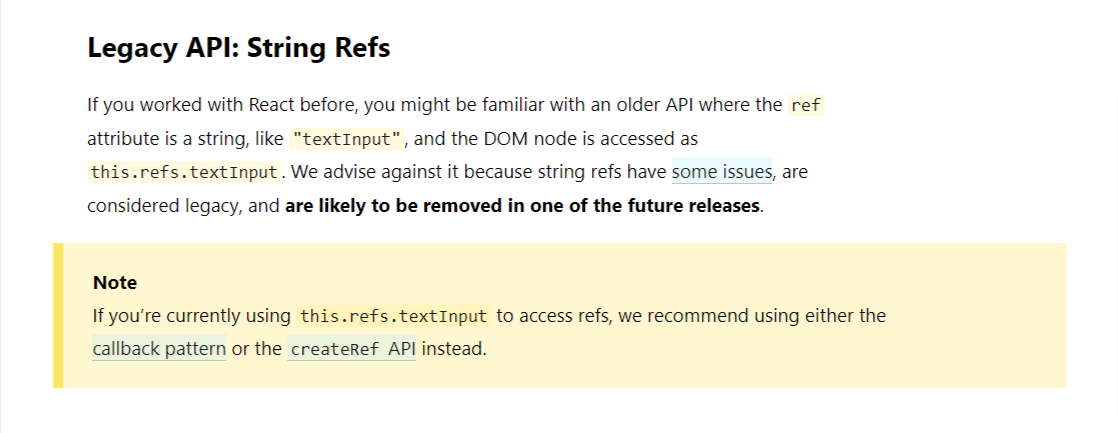 比方說,您有一個元件使用這樣的字串引用: ``` import { Component } from "react"; import { createRoot } from "react-dom/client"; class BlackMamba extends Component { componentDidMount() { this.refs.venom.focus(); } render() { return ( <> <h1>Black Mamba</h1> <input ref="venom" /> </> ); } } const root = createRoot(document.getElementById("root")); root.render(<BlackMamba />); ``` 您不會在控制台中看到任何錯誤。 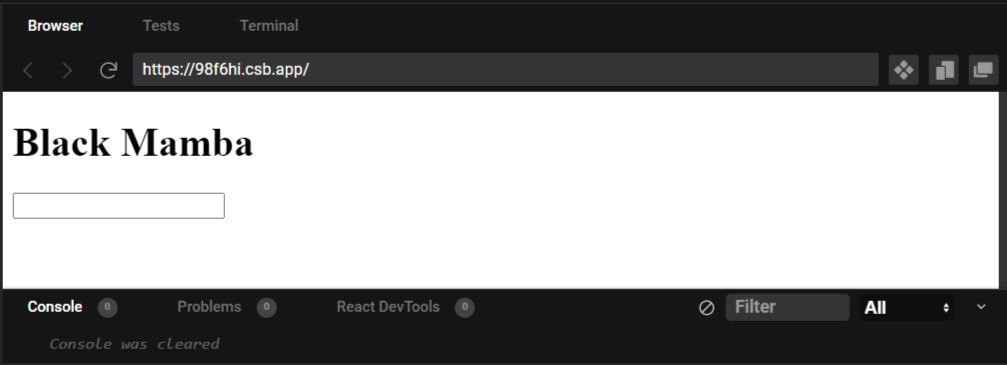 當您像這樣在您的應用中啟用“React.StrictMode”時: ``` import { StrictMode, Component } from "react"; import { createRoot } from "react-dom/client"; class BlackMamba extends Component { componentDidMount() { this.refs.venom.focus(); } render() { return ( <> <h1>Black Mamba</h1> <input ref="venom" /> </> ); } } const root = createRoot(document.getElementById("root")); root.render( <StrictMode> <BlackMamba /> </StrictMode> ); ``` 您會在控制台中看到如下所示的警告: > Warning: A string ref, "venom", has been found within a strict mode tree. String refs are a source of potential bugs and should be avoided. We recommend using useRef() or createRef() instead. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-string-ref 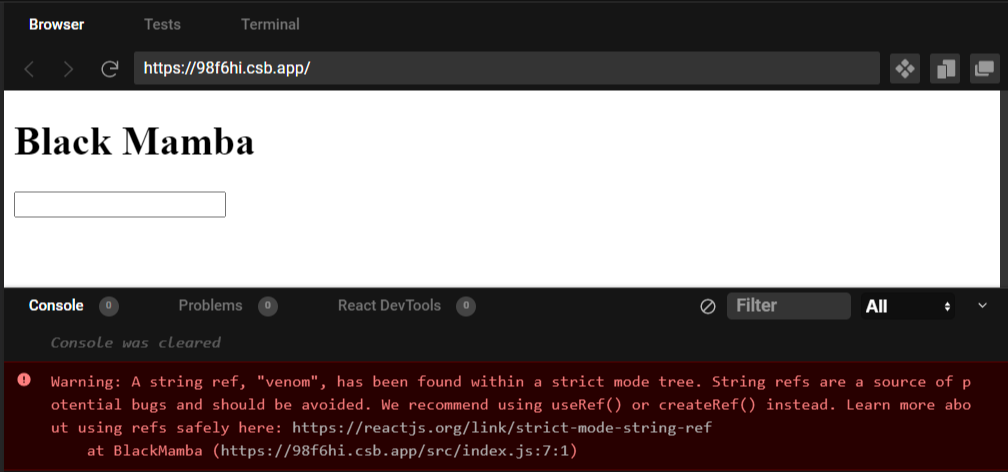 讓我們用 `createRef` API 修復它: ``` import { StrictMode, Component, createRef } from "react"; import { createRoot } from "react-dom/client"; class BlackMamba extends Component { constructor(props) { super(props); this.venom = createRef(); } componentDidMount() { this.venom.current.focus(); } render() { return ( <> <h1>Black Mamba</h1> <input ref={this.venom} /> </> ); } } const root = createRoot(document.getElementById("root")); root.render( <StrictMode> <BlackMamba /> </StrictMode> ); ``` 修理完成! 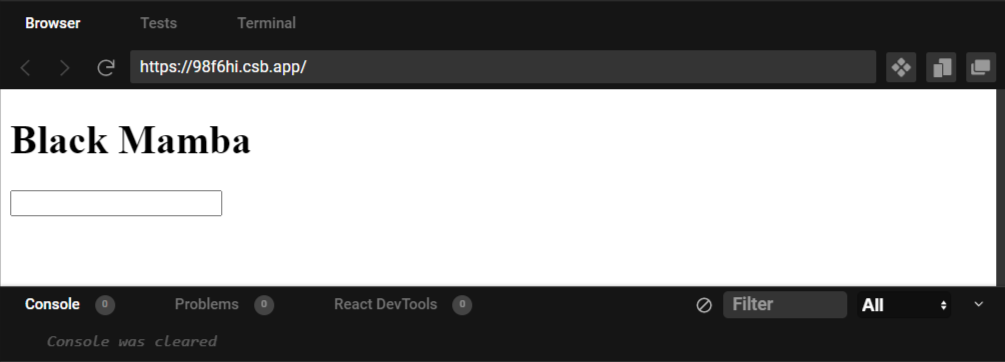 --- ## 過時的 Context API [舊版 Context API](https://legacy.reactjs.org/docs/legacy-context.html) 在 React 中已棄用,已被新的 Context API 取代。 React Strict Mode 可以幫助您檢測過時的 Context API 的任何使用情況,並鼓勵您切換到 [新 API](https://react.dev/reference/react/useContext) 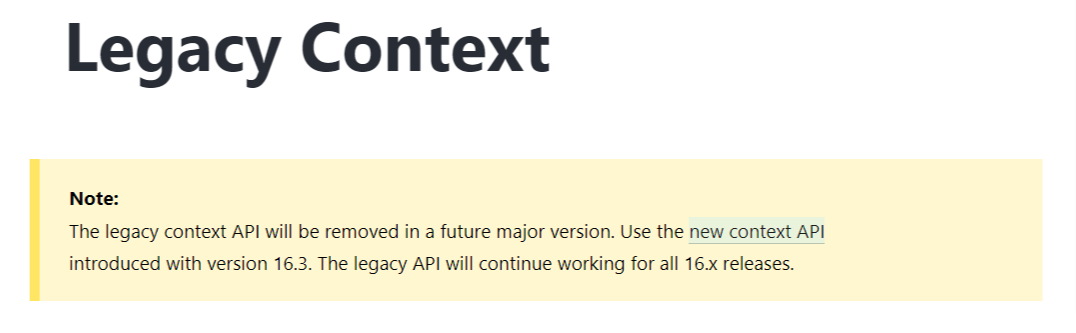 --- 如果您還沒有使用嚴格模式,那絕對值得使用看看! 以上簡單分享,希望對您有幫助!
你的轉職路上,還缺少一份自學作業包!寫完這幾包,直接拿作品去面試上班!
本論壇另有附設一個 LINE 新手發問&交流群組!歡迎加入討論!